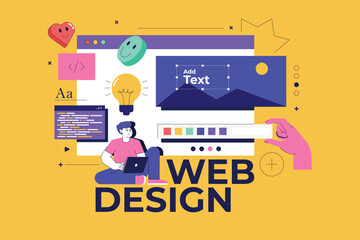
Understanding Errors in PHP
PHP errors can be classified into three main types:
-
Notices: Non-critical errors that do not halt script execution but indicate potential issues, such as accessing undefined variables.
-
Warnings: More severe than notices, warnings indicate potential problems that may cause issues but do not stop script execution.
-
Fatal Errors: Critical errors that halt script execution, such as calling undefined functions or accessing undefined classes.
Best Practices for PHP Error Handling
1. Enable Error Reporting
During development, ensure that error reporting is enabled to catch and address issues early. Set the following directives in your php.ini
file or at the beginning of your script:
error_reporting(E_ALL); ini_set('display_errors', 1);
2. Use Try-Catch Blocks
Enclose code that may throw exceptions or errors within try-catch blocks to handle them gracefully. This prevents fatal errors from halting script execution and allows you to handle errors in a controlled manner.
try { // Code that may throw exceptions or errors } catch (Exception $e) { // Handle the exception echo 'Caught exception: ', $e->getMessage(), "\n"; } catch (Error $e) { // Handle the error echo 'Caught error: ', $e->getMessage(), "\n"; }
3. Custom Error and Exception Handling
Define custom error and exception handlers to centralize error processing and provide consistent error responses. This allows you to log errors, display user-friendly messages, and gracefully handle errors throughout your application.
set_error_handler(function ($severity, $message, $file, $line) { throw new ErrorException($message, 0, $severity, $file, $line); }); set_exception_handler(function ($e) { // Log the exception error_log($e->getMessage()); // Display a user-friendly error message echo 'An unexpected error occurred. Please try again later.'; });
4. Logging Errors
Implement logging to record errors and exceptions for troubleshooting and debugging purposes. Use logging libraries like Monolog or write custom logging functions to log errors to files, databases, or external services.
// Example using Monolog use Monolog\Logger; use Monolog\Handler\StreamHandler; $log = new Logger('app'); $log->pushHandler(new StreamHandler('path/to/logfile.log', Logger::ERROR)); try { // Code that may throw exceptions or errors } catch (Exception $e) { // Log the exception $log->error($e->getMessage()); } catch (Error $e) { // Log the error $log->error($e->getMessage()); }
5. Graceful Error Responses
Provide informative and user-friendly error messages to users when errors occur. Avoid exposing sensitive information and provide clear instructions on how to resolve the issue or contact support.
try { // Code that may throw exceptions or errors } catch (Exception $e) { // Display a user-friendly error message echo 'An unexpected error occurred. Please try again later.'; } catch (Error $e) { // Display a user-friendly error message echo 'An unexpected error occurred. Please try again later.'; }
Conclusion
PHP error handling is an essential aspect of writing resilient and reliable code. By enabling error reporting, using try-catch blocks, implementing custom error and exception handlers, logging errors, and providing graceful error responses, you can ensure that your PHP applications handle errors gracefully and provide a positive user experience.
Embrace these best practices for PHP error handling to write more robust and resilient code that can withstand unexpected errors and edge cases. Remember to test your error handling mechanisms thoroughly to identify and address any potential issues before deploying your applications to production.