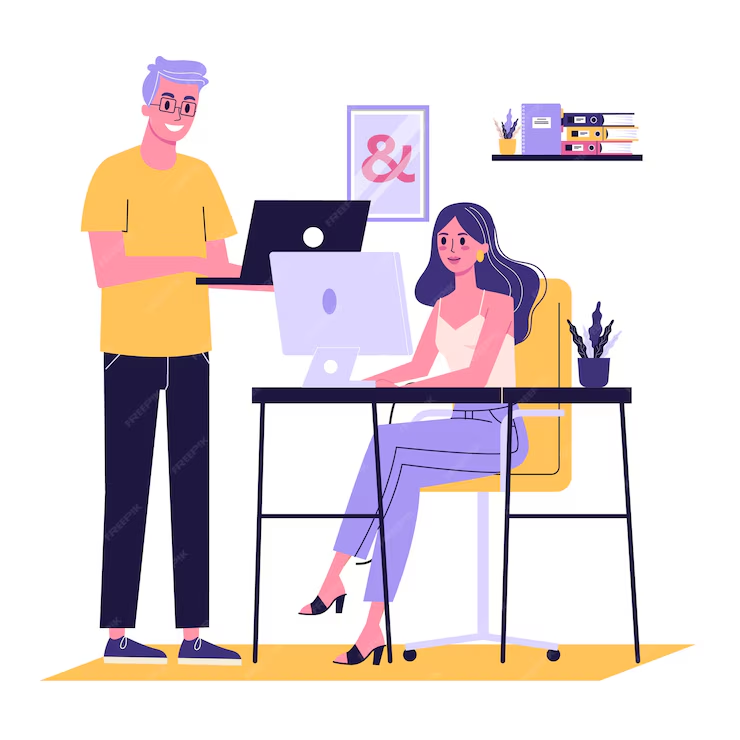
Understanding Mobile-First Design
Before diving into PHP, it’s essential to understand what mobile-first design entails. Mobile-first design prioritizes the mobile user experience over the desktop. This approach ensures that websites are fully functional and visually appealing on smaller screens before scaling up to larger devices. Key principles include:
- Responsive Design: Creating layouts that adapt to various screen sizes.
- Performance Optimization: Ensuring fast load times on mobile networks.
- User Experience: Simplifying navigation and interaction for touch interfaces.
Why PHP for Mobile-First Web Development?
PHP is a versatile language that offers several advantages for mobile-first web development:
- Server-Side Processing: PHP handles server-side logic, enabling dynamic content generation and efficient data management.
- Framework Support: Popular PHP frameworks like Laravel, Symfony, and CodeIgniter provide tools and libraries to streamline mobile-first development.
- Scalability: PHP scales easily to accommodate growing traffic and evolving features.
- Community and Resources: A vast community and extensive documentation make PHP an accessible and well-supported choice.
Key PHP Techniques for Mobile-First Development
1. Responsive Data Handling
Handling data responsively is crucial for mobile-first applications. PHP can dynamically serve different data formats and sizes based on the user’s device. For instance, you can use PHP to detect the device type and serve optimized images:
function serveImage($imagePath) { $deviceType = detectDevice(); if ($deviceType == 'mobile') { // Serve a smaller, optimized image for mobile return $imagePath . '_mobile.jpg'; } else { // Serve the standard image for desktop return $imagePath . '.jpg'; } } function detectDevice() { // Simple device detection logic if (preg_match('/Mobile|Android|iPhone|iPad/', $_SERVER['HTTP_USER_AGENT'])) { return 'mobile'; } else { return 'desktop'; } }
2. Efficient Caching Strategies
Caching is vital for performance, especially on mobile networks. PHP supports various caching mechanisms, such as:
- Opcode Caching: Using tools like OPcache to cache precompiled script bytecode.
- Data Caching: Leveraging solutions like Memcached or Redis to store frequently accessed data.
Implementing caching in PHP can significantly reduce load times and server load:
// Using Memcached for caching $memcached = new Memcached(); $memcached->addServer('localhost', 11211); $key = 'user_profile_' . $userId; $userProfile = $memcached->get($key); if ($userProfile === false) { // Fetch data from the database $userProfile = getUserProfileFromDatabase($userId); $memcached->set($key, $userProfile, 3600); // Cache for 1 hour } // Output user profile echo $userProfile;
3. Progressive Web Apps (PWAs)
Progressive Web Apps combine the best of web and mobile apps, providing a seamless user experience. PHP can serve as the backend for PWAs, handling data requests and user interactions. Key features of PWAs include:
- Service Workers: For offline functionality and background syncing.
- Responsive Design: Ensuring the app works on any device.
- App Shell Model: Delivering the basic UI shell quickly and loading content dynamically.
4. RESTful APIs
Building RESTful APIs with PHP allows for a decoupled architecture, where the frontend (often a JavaScript framework) and backend (PHP) interact seamlessly. This separation enhances the mobile-first approach by enabling the development of highly responsive and interactive mobile interfaces:
// Simple RESTful API endpoint in PHP header('Content-Type: application/json'); if ($_SERVER['REQUEST_METHOD'] == 'GET') { $data = getDataFromDatabase(); echo json_encode($data); } elseif ($_SERVER['REQUEST_METHOD'] == 'POST') { $input = json_decode(file_get_contents('php://input'), true); saveDataToDatabase($input); echo json_encode(['status' => 'success']); }
5. Utilizing PHP Frameworks
Frameworks like Laravel and Symfony offer built-in features that simplify mobile-first development:
- Blade (Laravel): A templating engine that facilitates the creation of responsive views.
- Twig (Symfony): Another powerful templating engine that supports responsive design principles.
- Eloquent ORM (Laravel): Simplifies database interactions, allowing for efficient data retrieval and manipulation.
Conclusion
PHP is a powerful ally in the quest for mobile-first web development. By leveraging its capabilities, from responsive data handling to efficient caching and API development, you can create web applications that are fast, scalable, and user-friendly on any device. Embracing the mobile-first approach with PHP not only meets the demands of today’s users but also future-proofs your applications for the evolving digital landscape. Start harnessing the power of PHP today to deliver outstanding mobile-first web experiences!